Playwright Mobile Testing: Tutorial & Best Practices
Automated testing is critical to modern software development, ensuring speed, quality, and reliability. Effective mobile testing has become essential in the mobile-first world, where mobile web usage is rapidly growing. Playwright, a powerful end-to-end automation tool, plays a role by offering a range of features tailored to mobile testing. Its ability to simulate mobile environments and handle real devices and emulators without additional drivers makes it particularly valuable for mobile automation strategies.
This article explores Playwright’s unique strengths for mobile web testing, including its cross-browser capabilities, performance optimization features, and powerful automation mechanisms. Prescriptive solutions are provided for common mobile testing pain points, like flakiness in automated tests. We also guide you through setting up Playwright for mobile testing, demonstrate how to integrate it into CI/CD pipelines, and cover advanced testing scenarios.
By the end of this article, you will understand how to implement Playwright for mobile automation to enhance your testing efficiency and deliver high-quality mobile experiences.
Summary of key Playwright mobile testing concepts
Core Playwright features for mobile web testing
Playwright offers features tailored for mobile web testing, making it an ideal tool for modern mobile development. With capabilities such as viewport simulation, cross-browser testing, and network condition emulation, Playwright enables developers to ensure consistent and reliable performance across various devices and platforms.
Mobile viewport simulation
Playwright excels at simulating mobile viewports, allowing for accurate testing of various screen sizes. It enables the emulation of devices such as iPhones, Android phones, and tablets, so user experiences are consistent across different devices. Playwright can also connect to real devices or emulators, allowing teams to test mobile web applications under real-world conditions.
Cross-browser testing capabilities
One of Playwright’s standout features is its ability to test across many browsers and devices. Beyond web browsers like Chrome, specific versions of mobile devices can be emulated, like the iPhone 13. This ensures that web applications behave consistently across various platforms. For mobile web testing, this cross-browser capability allows for broad compatibility testing without needing additional configurations, leading to uniform behavior across browsers.
Network condition simulation and offline mode testing
Playwright allows testers to simulate various network conditions, such as 3G, 4G, and offline modes. This helps check that mobile applications gracefully handle poor connectivity by showing proper error messages or gracefully degrading functionality.
Powerful selectors and auto-waiting mechanism
Playwright’s selectors, including CSS, XPath, and text selectors, simplify the automation process. Its auto-waiting mechanism ensures that tests don’t proceed until the necessary elements are ready, reducing test flakiness and improving reliability.
Combined with Playwright’s intelligent wait strategies, Qualiti’s platform allows AI to make real-time updates to test scripts and results, so tests stay up to date without manual intervention. This automation reduces flakiness and enhances test reliability, streamlining the process for engineering teams.
{{banner-large-dark-2="/banners"}}
Playwright mobile testing configuration
Playwright installation
Getting started with Playwright for mobile testing is straightforward. First, install Playwright via Node.js, and from there, configure it to emulate mobile devices by defining specific device viewports in the configuration file. This simple setup allows you to test your applications across various mobile devices without requiring complex configuration steps.
npx init playwright@latest
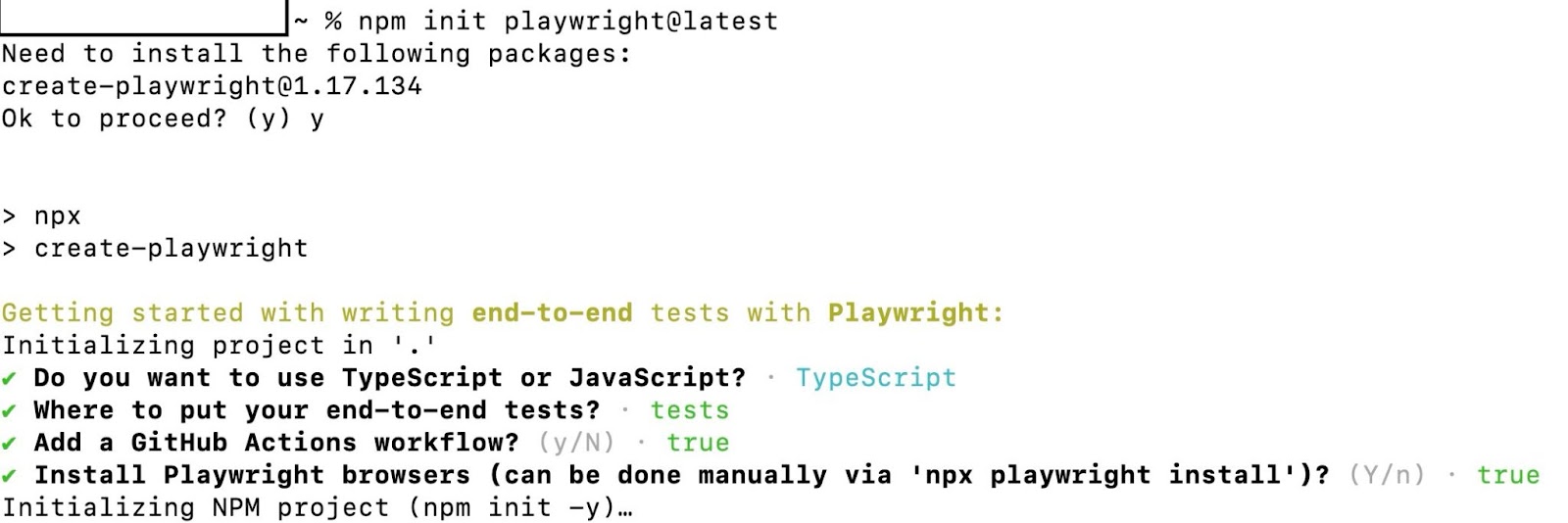
Configuring mobile viewports and emulated devices
Playwright allows testers to emulate mobile devices by configuring specific mobile viewports. For example, you can install an iPhone 12 simulation using Playwright’s built-in device configurations. This ensures that the application is tested in an environment that accurately mimics a real mobile device, giving you insights into how the app behaves across different screen sizes.
await browser.newContext({
...devices['iPhone 12'],
});
Handling Real Devices and Emulators
Playwright supports direct connections to real devices or emulators for more realistic mobile testing. Using the Android Debug Bridge (ADB), Playwright can connect to Android emulators or real devices, allowing tests to be executed in a real-world environment. This removes the need for additional software layers and allows for more accurate results when testing mobile web applications.
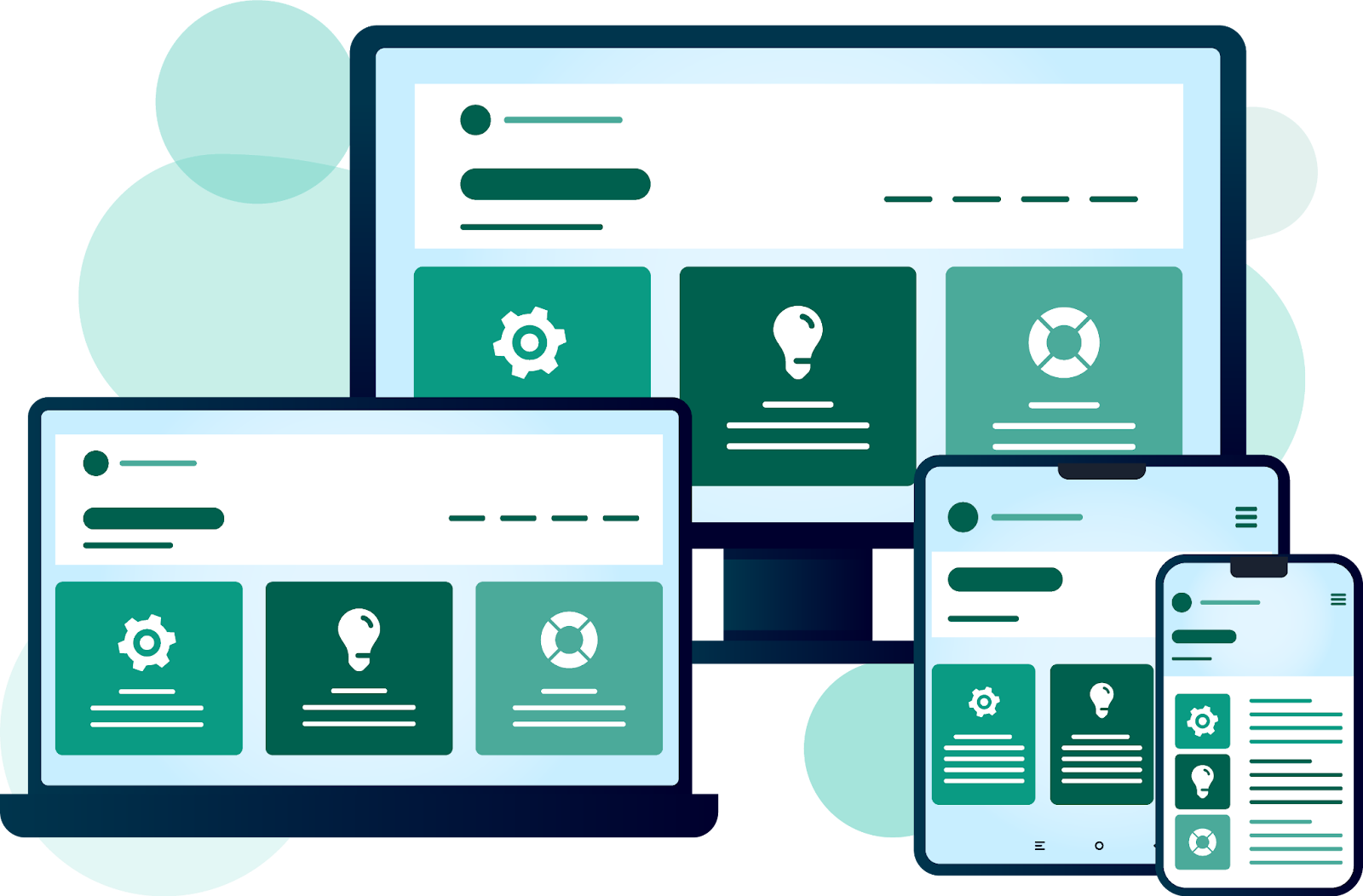
Note that while emulators provide convenience and speed for testing, they may not always reflect the exact behavior of real devices. Physical devices offer a more accurate representation of the user experience but come with added complexity and resource requirements. The right balance between emulators and real devices depends on the specific needs of the testing process.
Playwright performance testing for the mobile web
Playwright provides several tools and strategies to monitor and enhance the performance of mobile web applications. Below are some common techniques to streamline performance testing:
- Measuring page load times: Check load times by recording performance data during test execution to optimize real-user experiences.
const [response] = await Promise.all([
page.goto('https://example.com'),
page.waitForLoadState('load'),
]);
- Tab management and cache clearing: Close unused browser tabs and clear the browser cache after test execution to prevent performance slowdowns. This ensures efficient resource management and smoother mobile test runs by avoiding memory overload from accumulated tabs and cached data.
- Simulating network conditions: Test application behavior under various network conditions, such as offline mode or poor connectivity. Here’s how to emulate the browser’s network connection going offline:
await context.setOffline(true);
This checks that the app can gracefully handle network interruptions. For multi-step tests, the context can be set online again later in the test.
{{banner-small-3="/banners"}}
Integrating Playwright mobile testing into CI/CD pipelines
Seamlessly integrating Playwright mobile tests into CI/CD pipelines allows for automated testing at every stage of development. Key features include the following.
Automated testing in CI/CD
Integrating Playwright into CI/CD pipelines ensures that mobile tests are automatically executed during development. By setting up tools like GitHub Actions or CircleCI, you can automate mobile testing:
name: Playwright Tests
on: [push]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- run: npm install
- run: npx playwright test
The example above shows a basic GitHub Actions workflow. It first installs dependencies and then runs Playwright. A push event (when new code changes are uploaded to a repository) triggers it. This setup makes sure that mobile tests run seamlessly alongside every code change.
Parallel test execution
To speed up testing, Playwright supports parallel execution of tests across different devices and configurations. This can be very granular: Parallelism can be set at the root, project, or test level. Using parallelism ensures broad coverage and reduces the time needed for comprehensive mobile testing.
import { defineConfig } from '@playwright/test';
export default defineConfig({
fullyParallel: true,
});
Reporting and analyzing results
Playwright provides detailed reports and insights into test execution, helping teams quickly identify and resolve issues. Using tools like Playwright’s built-in reporters, you can generate HTML reports to track test outcomes.
CI integrations are built into the Qualiti platform, and new integrations can be set up during project creation.

Using Playwright in advanced mobile testing scenarios
Certain advanced scenarios require specialized testing methods to ensure a seamless user experience when testing mobile applications. Playwright supports robust testing features for handling these scenarios effectively, allowing developers to ensure smooth functionality across devices and conditions.
This table lists some key scenarios that Playwright can handle for advanced mobile testing.
Incorporating advanced mobile testing scenarios with Playwright ensures that apps perform seamlessly across devices and conditions. By handling real-world interactions like gestures, orientation changes, and managing pop-ups, Playwright helps developers catch issues early and maintain high-quality mobile experiences.
Best practices for Playwright mobile testing
Here are some important practices to make the most of mobile testing with Playwright:
- Prioritize testing critical features: Focus testing efforts on the most essential functions of your mobile application to be sure that core components work seamlessly.
- Optimize tests for stability: To ensure stable test results, use Playwright’s wait strategies, such as waitFor and waitForUrl. This helps reduce flakiness and ensures that elements are ready for interaction.
- Shift testing efforts to the left: “Shifting left” refers to testing as soon as possible in the development cycle. Incorporate mobile testing early in the development process to catch issues sooner, reducing the cost and time spent fixing bugs later in the cycle.
- Leverage notifications to create alerts: Integrate tools like Qualiti to establish notifications that alert engineers to critical issues during mobile testing. A proactive approach speeds up issue resolution and enhances overall testing efficiency.
Real-world examples
Example: Mobile login test script
A Playwright mobile login test ensures that the login flow works smoothly on mobile devices. A basic example might involve navigating to the login page, filling in the username and password fields, and verifying that the user is successfully logged in:
test('Mobile login test', async ({ page }) => {
await page.goto('https://example.com/login');
await page.fill('#username', 'user');
await page.fill('#password', 'password');
await page.click('button[type=submit]');
expect(page).toHaveText('Welcome');
});
Example: Handling browser tab management
Managing browser tabs is a common scenario in mobile testing. Playwright allows you to handle tabs effectively by opening links in new tabs and ensuring smooth transitions between them:
const [newPage] = await Promise.all([
context.waitForEvent('page'),
page.click('a[target=_blank]'),
]);
await newPage.close();
await page.bringToFront();
Verifying that browser tabs are managed efficiently adds an extra layer of coverage to mobile performance testing.
Qualiti’s approach to mobile testing automation
Qualiti enhances the mobile testing process by bringing Playwright’s robust automation capabilities into a streamlined, AI-powered platform. Unlike other tools that merely assist in writing tests, Qualiti goes further by automatically generating full test suites by analyzing your application’s content. This eliminates the need for extensive manual scripting, reduces flakiness, and improves test reliability. With minimal input, you can create and maintain comprehensive tests that adapt to changes in the application—saving time and keeping your testing aligned with your development efforts.

Errors are displayed visually with traces, replays, and metadata:
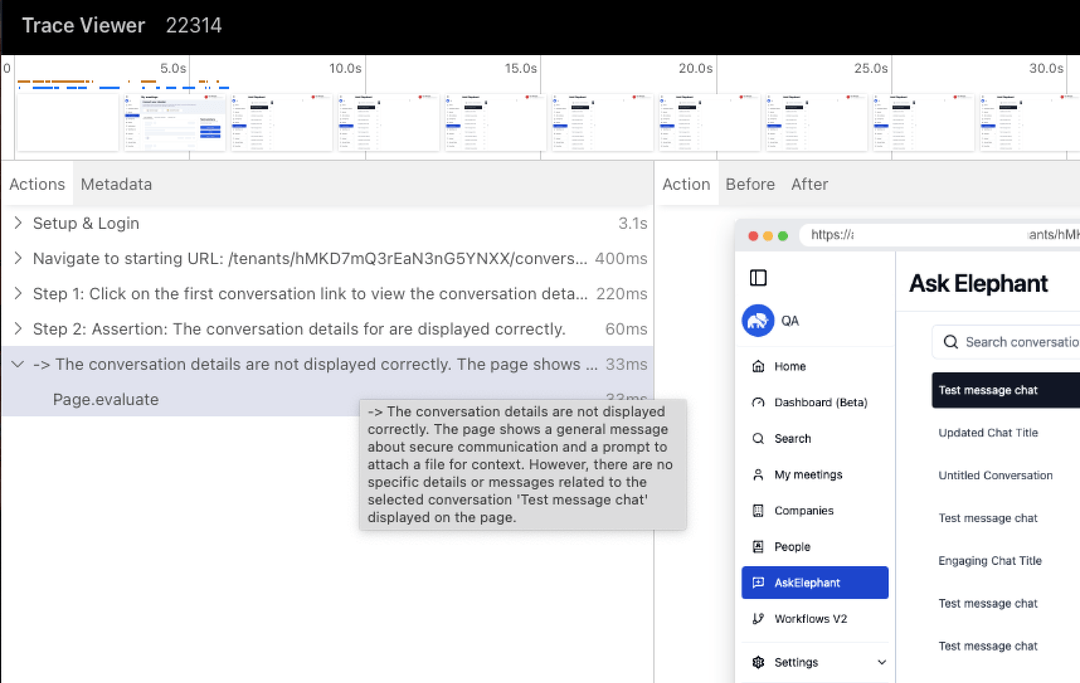
With Qualiti’s Playwright integration, Playwright’s strengths come together in a low-code, AI-driven workflow, helping teams streamline their processes and deliver high-quality results with less effort.
{{banner-small-4="/banners"}}
Conclusion
Mobile applications and mobile testing are both large, complex subjects. This guide introduced concepts such as parallelism, automation, cross-device coverage, and network simulations. Incorporating Playwright into testing strategies ensures that mobile applications meet the high-performance standards users expect in today’s mobile-first world. It offers robust capabilities for mobile testing, making it a top choice for modern app development.